If you're like me, many of the applications you build in Laravel have a similar Saas-type framework: user signup, user login, password reset, public sales page, logged-in dashboard, logout route, and a base Bootstrap style for when you're just getting started.
Laravel used to have a scaffold for this out of the box. It disappeared recently, to my great chagrin, but it's now back as an Artisan command:
make:auth
.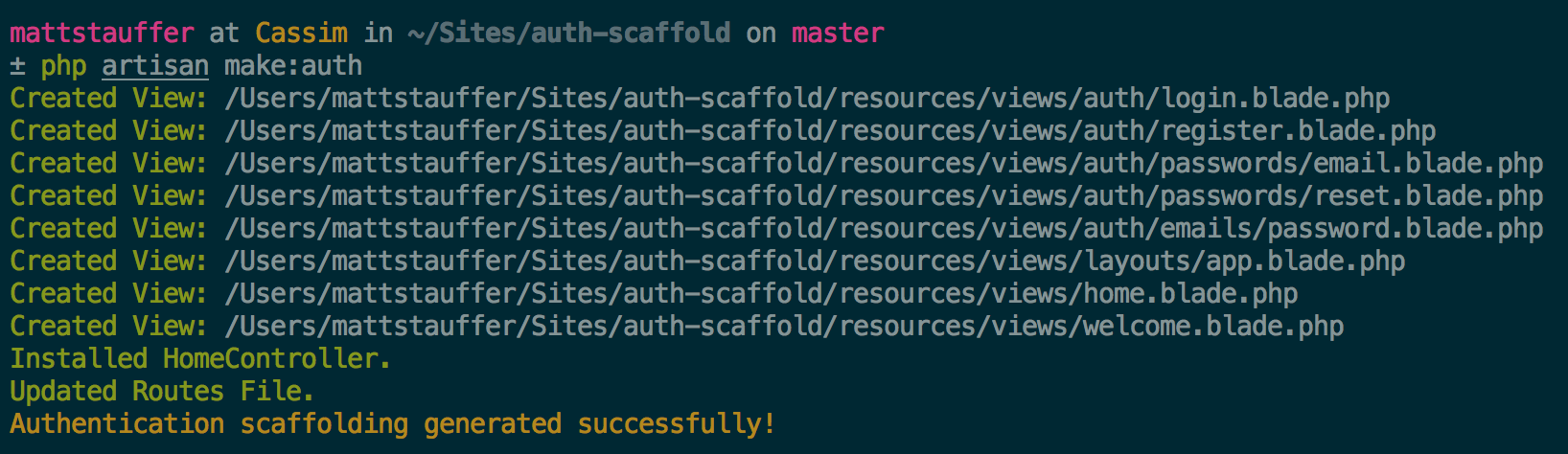
What does it provide? Let's dig in.
What changed? #
We have a layout (
resources/views/layouts/app.blade.php
) that is the core of this scaffold, and then a series of views that extend it:- welcome.blade.php - the public welcome page
- home.blade.php - the dashboard for logged-in users
- auth/login.blade.php - the login page
- auth/register.blade.php - the register/signup page
- auth/passwords/email.blade.php - the password reset confirmation page
- auth/passwords/reset.blade.php - the password reset prompt page
- auth/emails/password.blade.php - the password reset email
Our public page is still routed via
routes.php
:Route::get('/', function () {
return view('welcome');
});
And we now have a
HomeController
, which routes our dashboard:class HomeController extends Controller
{
/**
* Show the application dashboard.
*
* @return Response
*/
public function index()
{
return view('home');
}
}
This is of course routed in
routes.php
in the web
group. And notice that there's something else new there: The Route::auth()
method:Route::group(['middleware' => 'web'], function () {
Route::auth();
Route::get('/home', 'HomeController@index');
});
Route::auth() #
The
auth()
method is a shortcut to defining the following routes:// Authentication Routes...
$this->get('login', 'Auth\AuthController@showLoginForm');
$this->post('login', 'Auth\AuthController@login');
$this->get('logout', 'Auth\AuthController@logout');
// Registration Routes...
$this->get('register', 'Auth\AuthController@showRegistrationForm');
$this->post('register', 'Auth\AuthController@register');
// Password Reset Routes...
$this->get('password/reset/{token?}', 'Auth\PasswordController@showResetForm');
$this->post('password/email', 'Auth\PasswordController@sendResetLinkEmail');
$this->post('password/reset', 'Auth\PasswordController@reset');
The frontend #
Now let's take a look at what we get in the browser:
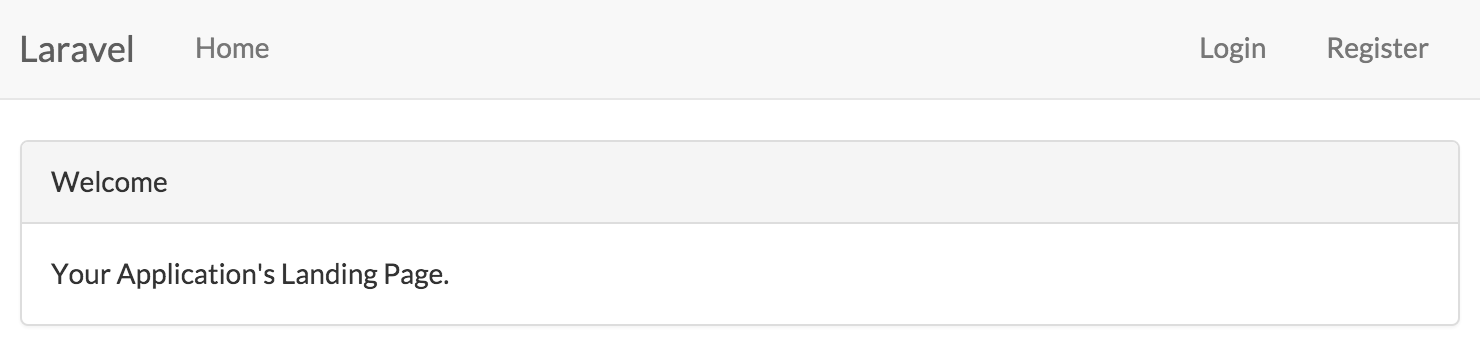
As you can see we have Bootstrap CSS, a basic Bootstrap app layout, and helpful to our basic auth actions.
App.blade.php #
So what does this master layout look like?
We get FontAwesome, the Lato font, Bootstrap CSS, a basic hamburger-on-mobile responsive layout, jQuery, Bootstrap JS, and placeholders that are commented out for the default output CSS and JS files if you choose to use Elixir.
We also have a top nav that links us home, and links guests to either login or register, and links authenticated users to log out.
Conclusion #
That's it! It's not anything complex, but it's 30-60 minutes of typing that you just saved on every app that needs it.
from : https://mattstauffer.co/blog/the-auth-scaffold-in-laravel-5-2